After logging in to Chat, users can send the following types of messages to a peer user, a chat group, or a chat room:
The Chat message feature is language agnostic. End users can send messages in any language, as long as their devices support input in that language.
This page shows how to implement sending and receiving these messages using the Chat SDK.
This section shows how to implement sending and receiving the various types of messages.
Use the ChatMessage
class to create a text message, and send the message.
_19// Call createTextSendMessage to create a text message. Set content as the content of the text message, and toChatUsernames the user ID of the message recipient.
_19ChatMessage message = ChatMessage.createTxtSendMessage(content, toChatUsername);
_19// Set the message type using the MessageType attribute in Message.
_19// You can set `MessageType` as `Chat`, `Group`, or `Room`, which indicates whether to send the message to a peer user, a chat group, or a chat room.
_19message.setChatType(ChatType.GroupChat);
_19// Calls setMessageStatusCallback to set the callback instance to get the status of messaging sending. You can update the status of messages in this callback, for example, adding a tip when the message sending fails.
_19 message.setMessageStatusCallback(new CallBack() {
_19 public void onSuccess() {
_19 showToast("Message sending succeeds");
_19 public void onError(int code, String error) {
_19 showToast("Message sending fails");
_19ChatClient.getInstance().chatManager().sendMessage(message);
You can use MessageListener
to listen for message events. You can add multiple MessageListener
s to listen for multiple events. When you no longer listen for an event, ensure that you remove the listener.
When a message arrives, the recipient receives an OnMessageReceived callback. Each callback contains one or more messages. You can traverse the message list, and parse and render these messages in this callback.
_11MessageListener msgListener = new MessageListener()
_11 // Traverse the message list, and parse and render the messages.
_11 public void onMessageReceived(List<ChatMessage> messages)
_11ChatClient.getInstance().chatManager().addMessageListener(msgListener);
_11ChatClient.getInstance().chatManager().removeMessageListener(msgListener);
Two minutes after a user sends a message, this user can withdraw it. Contact support@agora.io if you want to adjust the time limit.
_7 ChatClient.getInstance().chatManager().recallMessage(message);
_7 EMLog.d("TAG", "Recalling message succeeds");
_7} catch (ChatException e) {
_7 EMLog.e("TAG", "Recalling message fails: "+e.getDescription());
You can also use onMessageRecalled to listen for the message recall state:
_4 * Occurs when a received message is recalled.
_4void onMessageRecalled(List<ChatMessage> messages);
Voice, image, video, and file messages are essentially attachment messages. This section introduces how to send these types of messages.
Before sending a voice message, you should implement audio recording on the app level, which provides the URI and duration of the recorded audio file.
Refer to the following code example to create and send a voice message:
_6// Set voiceUri as the local URI of the audio file, and duration as the length of the file in seconds.
_6ChatMessage message = ChatMessage.createVoiceSendMessage(voiceUri, duration, toChatUsername);
_6// Sets the chat type as one-to-one chat, group chat, or chatroom.
_6if (chatType == CHATTYPE_GROUP)
_6 message.setChatType(ChatType.GroupChat);
_6ChatClient.getInstance().chatManager().sendMessage(message);
When the recipient receives the message, refer to the following code example to get the audio file:
_5VoiceMessageBody voiceBody = (VoiceMessageBody) msg.getBody();
_5// Retrieves the URL of the audio file on the server.
_5String voiceRemoteUrl = voiceBody.getRemoteUrl();
_5// Retrieves the URI if the audio file on the local device.
_5Uri voiceLocalUri = voiceBody.getLocalUri();
By default, the SDK compresses the image file before sending it. To send the original file, you can set original
as true
.
Refer to the following code example to create and send an image message:
_6// Set imageUri as the URI of the image file on the local device. false means not to send the original image. The SDK compresses image files that exceeds 100K before sending them.
_6ChatMessage message = ChatMessage.createImageSendMessage(imageUri, false, toChatUsername);
_6// Sets the chat type as one-to-one chat, group chat, or chatroom.
_6if (chatType == CHATTYPE_GROUP)
_6 message.setChatType(ChatType.GroupChat);
_6ChatClient.getInstance().chatManager().sendMessage(message);
When the recipient receives the message, refer to the following code example to get the thumbnail and attachment file of the image message:
_10// Retrieves the thumbnail and attachment of the image file.
_10ImageMessageBody imgBody = (ImageMessageBody) message.getBody();
_10// Retrieves the image file the server.
_10String imgRemoteUrl = imgBody.getRemoteUrl();
_10// Retrieves the image thumbnail from the server.
_10String thumbnailUrl = imgBody.getThumbnailUrl();
_10// Retrieves the URI of the image file on the local device.
_10Uri imgLocalUri = imgBody.getLocalUri();
_10// Retrieves the URI of the image thumbnail on the local device.
_10Uri thumbnailLocalUri = imgBody.thumbnailLocalUri();
If ChatClient.getInstance().getOptions().getAutodownloadThumbnail()
is set as true
on the recipient's client, the SDK automatically downloads the thumbnail after receiving the message. If not, you need to call ChatClient.getInstance().chatManager().downloadThumbnail(message)
to download the thumbnail and get the path from the thumbnailLocalUri
member in messageBody
.
Before sending a video message, you should implement video capturing on the app level, which provides the duration of the captured video file.
Refer to the following code example to create and send a video message:
_8String thumbPath = getThumbPath(videoUri);
_8// Set videoUri as the URI of the video file on the local device and videoLength as the length of the file in seconds,
_8// Set thumbPath as the video capturing local path.
_8ChatMessage message = ChatMessage.createVideoSendMessage(videoUri, thumbPath, videoLength, toChatUsername);
_8// Set the chat type as one-to-one chat, group chat, or chatroom.
_8if (chatType == CHATTYPE_GROUP)
_8message.setChatType(ChatType.GroupChat);
_8ChatClient.getInstance().chatManager().sendMessage(message);
By default, when the recipient receives the message, the SDK downloads the thumbnail of the video message.
If you do not want the SDK to automatically download the video thumbnail, set ChatClient.getInstance().getOptions().setAutodownloadThumbnail
as false
, and to download the thumbnail, you need to call ChatClient.getInstance().chatManager().downloadThumbnail(message)
, and get the path of the thumbnail from the thumbnailLocalUri
member in messageBody
.
To download the actual video file, call ChatClient.getInstance().chatManager().downloadAttachment(message)
, and get the path of the video file from the getLocalUri
member in messageBody
.
_31// If you received a message with video attachment, you need to download the attachment before you open it.
_31if (message.getType() == ChatMessage.Type.VIDEO)
_31 VideoMessageBody messageBody = (VideoMessageBody)message.getBody();
_31 // Get the URL of the video on the server.
_31 String videoRemoteUrl = messageBody.getRemoteUrl();
_31 CallBack callBack = new CallBack()
_31 public void onSuccess()
_31 // Download successfully
_31 public void onProgress(int progress, String status)
_31 public void onError(int code, String error)
_31 // Set Callback to know whether the download is finished.
_31 message.setMessageStatusCallback(callBack);
_31 // Download the video.
_31 ChatClient.getInstance().chatManager().downloadAttachment(message);
_31 // After the download finishes, get the URI of the local file.
_31 Uri videoLocalUri = messageBody.getLocalUri();
Refer to the following code example to create, send, and receive a file message:
_5// Set fileLocalUri as the URI of the file message on the local device.
_5ChatMessage message = ChatMessage.createFileSendMessage(fileLocalUri, toChatUsername);
_5// Sets the chat type as one-to-one chat, group chat, or chatroom.
_5if (chatType == CHATTYPE_GROUP) message.setChatType(ChatType.GroupChat);
_5ChatClient.getInstance().chatManager().sendMessage(message);
While sending a file message, refer to the following sample code to get the progress for uploading the attachment file:
_12// Calls setMessageStatusCallback to set the callback instance to listen for the state of messaging sending. You can update the message states in this callback.
_12message.setMessageStatusCallback(new CallBack() {
_12 public void onSuccess() {
_12 // Send message success
_12 public void onError(int code, String error) {
_12 // Send message failed
_12ChatClient.getInstance().chatManager().sendMessage(message);
When the recipient receives the message, call the downloadAttachment
method and then refer to the following code example to get the attachment file:
_25CallBack callBack = new CallBack() {
_25 public void onSuccess() {
_25 // Download successfully
_25 public void onProgress(int progress, String status) {
_25 public void onError(int code, String error) {
_25// Set Callback to know whether the download is finished.
_25message.setMessageStatusCallback(callBack);
_25// Download the video.
_25ChatClient.getInstance().chatManager().downloadAttachment(message);
_25// After the download finishes, get the URI of the local file.
_25NormalFileMessageBody fileMessageBody = (NormalFileMessageBody) message.getBody();
_25// Retrieves the file from the server.
_25String fileRemoteUrl = fileMessageBody.getRemoteUrl();
_25// Retrieves the file from the local device.
_25Uri fileLocalUri = fileMessageBody.getLocalUri();
To send and receive a location message, you need to integrate a third-party map service provider. When sending a location message, you get the longitude and latitude information of the location from the map service provider; when receiving a location message, you extract the received longitude and latitude information and displays the location on the third-party map.
_7// Sets the latitude and longitude information of the address.
_7ChatMessage message = ChatMessage.createLocationSendMessage(latitude, longitude, locationAddress, toChatUsername);
_7// Sets the latitude and longitude information of the address.
_7ChatMessage message = ChatMessage.createLocationSendMessage(latitude, longitude, locationAddress, toChatUsername);
_7// Sets the chat type as one-to-one chat, group chat, or chatroom.
_7if (chatType == CHATTYPE_GROUP) message.setChatType(ChatType.GroupChat);
_7ChatClient.getInstance().chatManager().sendMessage(message);
CMD messages are command messages that instruct a specified user to take a certain action. The recipient deals with the command messages themselves.
- CMD messages are not stored in the local database.
- Actions beginning with
em_
and easemob::
are internal fields. Do not use them.
_12ChatMessage cmdMsg = ChatMessage.createSendMessage(ChatMessage.Type.CMD);
_12// Sets the chat type as one-to-one chat, group chat, or chat room
_12cmdMsg.setChatType(ChatType.GroupChat)String action="action1";
_12// You can customize the action
_12CmdMessageBody cmdBody = new CmdMessageBody(action);String toUsername = "test1";
_12ChatMessage cmdMsg = ChatMessage.createSendMessage(ChatMessage.Type.CMD);
_12// Sets the chat type as one-to-one chat, group chat, or chat room
_12cmdMsg.setChatType(ChatType.GroupChat)String action="action1";
_12// You can customize the action
_12CmdMessageBody cmdBody = new CmdMessageBody(action);String toUsername = "test1";
_12// Specify a username to send the CMD message.
_12cmdMsg.setTo(toUsername);cmdMsg.addBody(cmdBody); ChatClient.getInstance().chatManager().sendMessage(cmdMsg);
To notify the recipient that a CMD message is received, use a separate delegate so that users can deal with the message differently.
_11MessageListener msgListener = new MessageListener()
_11 // Occurs when the message is received
_11 public void onMessageReceived(List<ChatMessage> messages) {
_11 // Occurs when a CMD message is received
_11 public void onCmdMessageReceived(List<ChatMessage> messages) {
Typing indicators signify when other users are typing a message. This feature enables users to communicate efficiently and sets users' expectations about new interactions in a chat app. You can implement typing indicators via CMD messages.
The following diagram explains how typing indicators work.
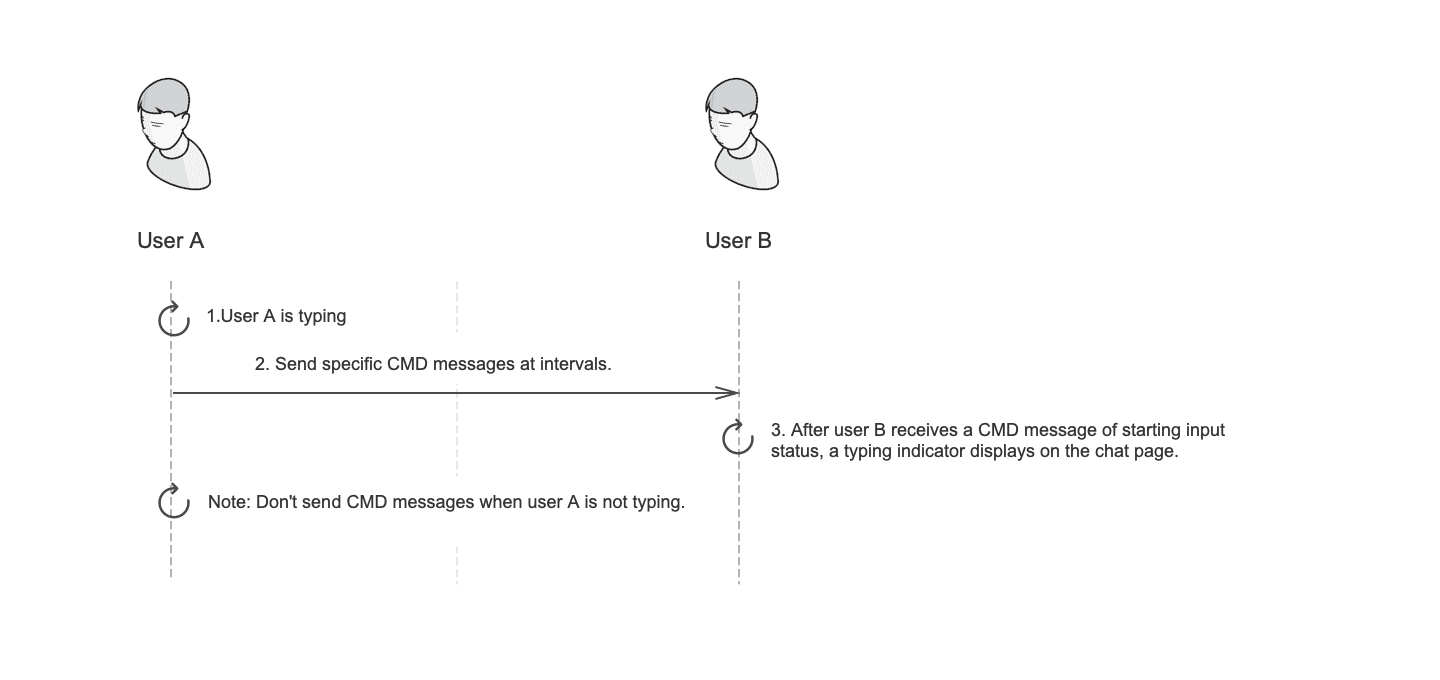
Listen for the input status of user A. Once there is text input, the input status is sent to user B through a command message, and user B receives the input status that indicates that user A is typing.
- User A sends a message to user B indicating the starting input status.
- After receiving the message, if user B is currently on the chat page of with user A, the typing indicator of user A is displayed.
- If user B receives the ending input status of user A or have not received from user A again after a few seconds, the typing indicator is automatically canceled.
Note:
User A can set the sending interval between CMD messages as needed.
The following code example shows how to send a CMD message of the input status.
_16EditText.addTextChangedListener(new TextWatcher() {
_16 public void onTextChanged(CharSequence s, int start, int before, int count) {
_16 sendCmdMessage(ACTION_TYPING_BEGIN);
_16public void sendCmdMessage(String action) {
_16 ChatMessage beginMsg = ChatMessage.createSendMessage(ChatMessage.Type.CMD);
_16 CmdMessageBody body = new CmdMessageBody(action);
_16 // Only deliver this CMD msg to online users.
_16 body.deliverOnlineOnly(true);
_16 beginMsg.addBody(body);
_16 beginMsg.setTo(toChatUsername);
_16 ChatClient.getInstance().chatManager().sendMessage(beginMsg);
The following code example shows how to receive and parse a CMD message of the input status.
_24ChatManager.getInstance().addMessageListener(new MessageListener() {
_24 public void onCmdMessageReceived(List<ChatMessage> messages) {
_24 for (final ChatMessage msg : messages) {
_24 final CmdMessageBody body = (CmdMessageBody) msg.getBody();
_24 EMLog.i(TAG, "Receive cmd message: " + body.action() + " - " + body.isDeliverOnlineOnly());
_24 EaseThreadManager.getInstance().runOnMainThread(() -> {
_24 if(TextUtils.equals(msg.getFrom(), conversationId)) {
_24 if (TextUtils.equals(action, EaseChatLayout.ACTION_TYPING_BEGIN)) {
_24 binding.subTitle.setText(getString(R.string.alert_during_typing));
_24 binding.subTitle.setVisibility(View.VISIBLE);
_24 } else if (TextUtils.equals(action, EaseChatLayout.ACTION_TYPING_END)) {
_24 if(typingHandler != null) {
_24 typingHandler.removeMessages(MSG_OTHER_TYPING_END);
_24 typingHandler.sendEmptyMessageDelayed(MSG_OTHER_TYPING_END, OTHER_TYPING_SHOW_TIME);
Custom messages are self-defined key-value pairs that include the message type and the message content.
The following code example shows how to create and send a customized message:
_12ChatMessage customMessage = ChatMessage.createSendMessage(ChatMessage.Type.CUSTOM);
_12// Set event as the customized message type, for example, gift.
_12String event = "gift";
_12CustomMessageBody customBody = new CustomMessageBody(event);
_12// The data type of params is Map<String, String>.
_12customBody.setParams(params);
_12customMessage.addBody(customBody);
_12// Specifies the user ID to receive the message, as Chat ID, chat group ID, or chat room ID.
_12customMessage.setTo(to);
_12// Sets the chat type as one-to-one chat, group chat, or chat room
_12customMessage.setChatType(chatType);
_12ChatClient.getInstance().chatManager().sendMessage(customMessage);
If the message types listed above do not meet your requirements, you can use message extensions to add attributes to the message. This can be applied in more complicated messaging scenarios.
_9ChatMessage message = ChatMessage.createTextSendMessage(content, toChatUsername);
_9// Adds message attributes.
_9message.setAttribute("attribute1", "value");
_9message.setAttribute("attribute2", true);
_9ChatClient.getInstance().chatManager().sendMessage(message);
_9// Retrieves the message attributes when receiving the message.
_9String attribute1 = message.getStringAttribute("attribute1",null);
_9boolean attribute2 = message.getBooleanAttribute("attribute2", false);
After implementing sending and receiving messages, you can refer to the following documents to add more messaging functionalities to your app: